Member-only story
10 UIView Extensions Every iOS Developer Should Know
If you often work with UIView, you’ll definitely understand why this extension is useful!

We often end up writing the same UIView
code over and over again in different projects.
Whether it’s adding shadows and rounded corners or handling custom animations, these repetitive tasks can make our code messy and slow us down.
That’s where UIView
extensions come in handy!
Explore 10 powerful UIView
extensions that can help simplify development process, and give iOS apps a more look better.
1. Adding Multiple Subviews at Once
How many times have you written multiple addSubview
calls one after another?
With this simple extension, you can add multiple subviews in just one function call!
extension UIView {
// Adding Multiple Subviews at Once
func addSubviews(_ views: UIView...) {
views.forEach { addSubview($0) }
}
}
✦ How to use:
view.addSubviews(titleLabel, imageView, descriptionLabel, actionButton)
So, with this extension, your code will be shorter and reduce the need to repeatedly write .addSubview
.
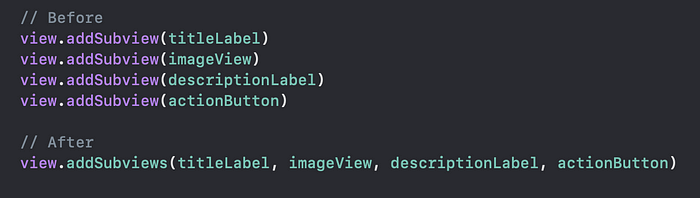
2. Pinning a View to its Superview
Setting up constraints to pin a view to its superview’s edges is something we do often.
This extension makes it easier by allowing customizable insets.
extension UIView {
// Pinning a View to its Superview
func pinToSuperview(top: CGFloat = 0, left: CGFloat = 0, bottom: CGFloat = 0, right: CGFloat = 0) {
guard let superview = superview else {
print("Error: superview does not exist")
return
}
translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activate([
topAnchor.constraint(equalTo: superview.topAnchor, constant: top),
leftAnchor.constraint(equalTo: superview.leftAnchor, constant: left)…